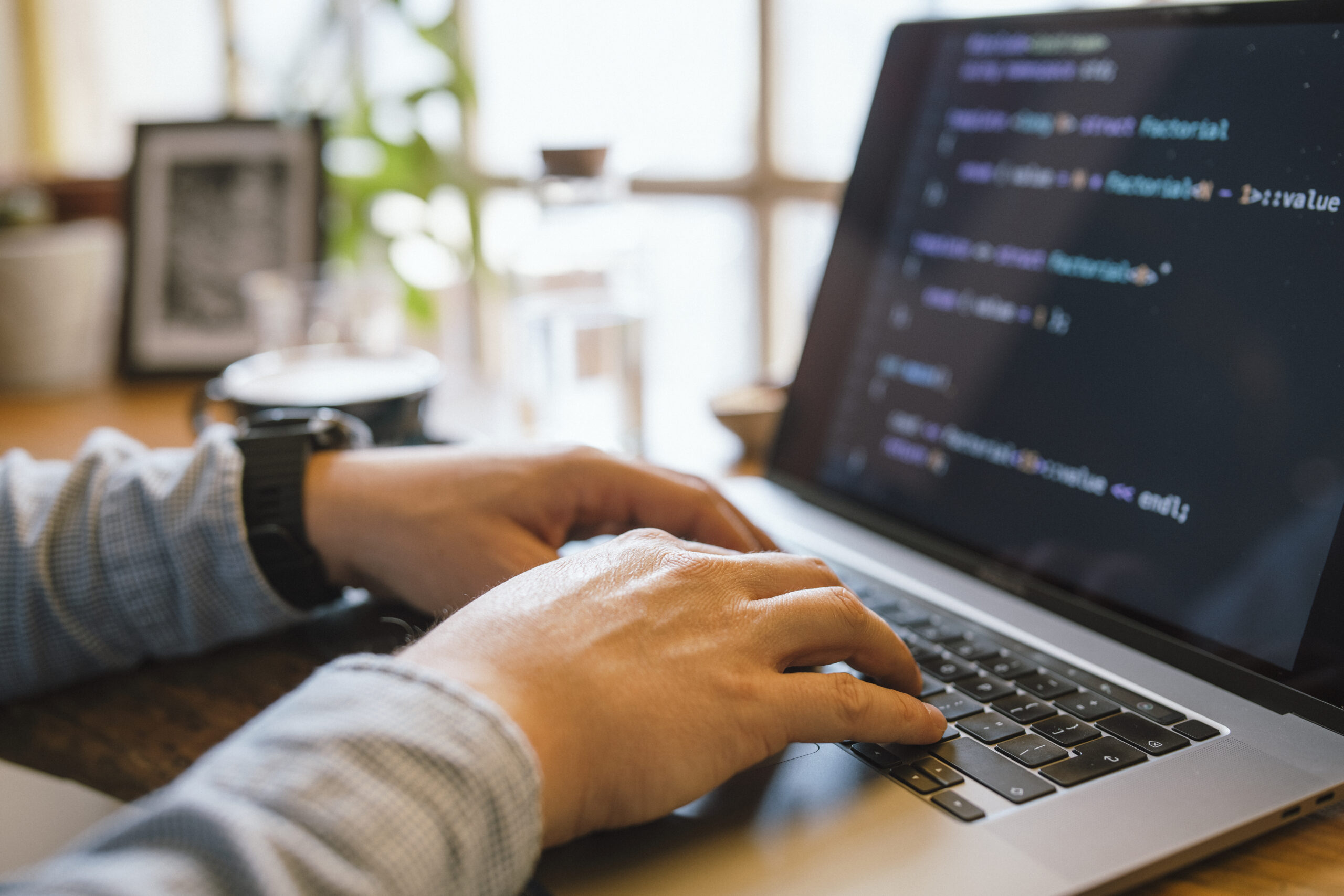
Debugging is Just about the most critical — however frequently disregarded — techniques inside of a developer’s toolkit. It's not just about fixing damaged code; it’s about knowledge how and why matters go wrong, and learning to Believe methodically to solve problems efficiently. Regardless of whether you're a newbie or perhaps a seasoned developer, sharpening your debugging expertise can help save several hours of annoyance and considerably transform your productiveness. Here's several strategies that will help builders stage up their debugging recreation by me, Gustavo Woltmann.
Master Your Equipment
One of many quickest ways builders can elevate their debugging techniques is by mastering the equipment they use everyday. Though producing code is a single Portion of development, recognizing tips on how to connect with it properly in the course of execution is equally significant. Modern day improvement environments arrive Geared up with strong debugging capabilities — but lots of developers only scratch the surface of what these tools can perform.
Get, for example, an Built-in Improvement Ecosystem (IDE) like Visible Studio Code, IntelliJ, or Eclipse. These equipment permit you to established breakpoints, inspect the value of variables at runtime, action via code line by line, and perhaps modify code over the fly. When employed correctly, they Enable you to notice specifically how your code behaves during execution, that's priceless for monitoring down elusive bugs.
Browser developer resources, for instance Chrome DevTools, are indispensable for front-conclude builders. They help you inspect the DOM, keep track of community requests, perspective actual-time general performance metrics, and debug JavaScript inside the browser. Mastering the console, resources, and community tabs can turn aggravating UI challenges into manageable duties.
For backend or process-degree builders, applications like GDB (GNU Debugger), Valgrind, or LLDB supply deep Regulate over working procedures and memory management. Understanding these instruments may have a steeper Studying curve but pays off when debugging functionality challenges, memory leaks, or segmentation faults.
Beyond your IDE or debugger, develop into snug with version Handle programs like Git to be aware of code history, come across the precise instant bugs were introduced, and isolate problematic modifications.
Eventually, mastering your equipment means going beyond default settings and shortcuts — it’s about creating an intimate understanding of your advancement natural environment to make sure that when issues crop up, you’re not lost in the dark. The greater you know your equipment, the more time you are able to invest solving the actual issue as an alternative to fumbling by the method.
Reproduce the challenge
The most essential — and sometimes ignored — methods in powerful debugging is reproducing the challenge. Ahead of jumping into the code or earning guesses, builders need to produce a reliable natural environment or situation exactly where the bug reliably seems. Devoid of reproducibility, correcting a bug gets a recreation of likelihood, frequently bringing about squandered time and fragile code alterations.
The initial step in reproducing a difficulty is gathering just as much context as you can. Ask issues like: What actions resulted in the issue? Which natural environment was it in — advancement, staging, or output? Are there any logs, screenshots, or error messages? The greater depth you have, the easier it will become to isolate the exact disorders beneath which the bug takes place.
As soon as you’ve gathered more than enough data, try to recreate the challenge in your local setting. This could signify inputting the identical details, simulating equivalent person interactions, or mimicking method states. If The difficulty seems intermittently, contemplate creating automatic checks that replicate the edge scenarios or state transitions concerned. These assessments not only enable expose the issue but in addition reduce regressions Later on.
Often, The difficulty might be ecosystem-certain — it would materialize only on particular working programs, browsers, or under unique configurations. Using resources like virtual devices, containerization (e.g., Docker), or cross-browser tests platforms is usually instrumental in replicating such bugs.
Reproducing the trouble isn’t just a step — it’s a attitude. It calls for endurance, observation, in addition to a methodical solution. But once you can persistently recreate the bug, you might be now midway to correcting it. Which has a reproducible state of affairs, you can use your debugging tools much more efficiently, examination likely fixes safely and securely, and converse far more Plainly with the team or customers. It turns an abstract criticism right into a concrete problem — and that’s in which developers thrive.
Go through and Realize the Error Messages
Error messages will often be the most beneficial clues a developer has when a little something goes Incorrect. Instead of looking at them as disheartening interruptions, builders need to find out to treat mistake messages as immediate communications within the technique. They typically let you know exactly what transpired, wherever it took place, and from time to time even why it occurred — if you know the way to interpret them.
Start out by looking through the message diligently and in complete. Several builders, particularly when below time tension, look at the very first line and straight away start off generating assumptions. But deeper from the error stack or logs may perhaps lie the real root cause. Don’t just duplicate and paste error messages into search engines like google — browse and realize them first.
Split the mistake down into areas. Is it a syntax mistake, a runtime exception, or possibly a logic mistake? Does it issue to a particular file and line range? What module or perform activated it? These concerns can tutorial your investigation and position you towards the accountable code.
It’s also handy to know the terminology with the programming language or framework you’re utilizing. Mistake messages in languages like Python, JavaScript, or Java normally observe predictable patterns, and Finding out to acknowledge these can significantly hasten your debugging process.
Some mistakes are obscure or generic, and in Individuals scenarios, it’s essential to examine the context where the mistake occurred. Check out similar log entries, input values, and up to date improvements in the codebase.
Don’t neglect compiler or linter warnings both. These generally precede larger problems and supply hints about opportunity bugs.
Ultimately, error messages usually are not your enemies—they’re your guides. Finding out to interpret them the right way turns chaos into clarity, helping you pinpoint problems more quickly, cut down debugging time, and become a more effective and assured developer.
Use Logging Properly
Logging is The most strong instruments in a very developer’s debugging toolkit. When employed efficiently, it provides actual-time insights into how an application behaves, aiding you fully grasp what’s occurring beneath the hood while not having to pause execution or move in the code line by line.
A very good logging system starts off with figuring out what to log and at what stage. Widespread logging stages involve DEBUG, Facts, Alert, ERROR, and FATAL. Use DEBUG for detailed diagnostic information all through enhancement, Details for typical gatherings (like prosperous start off-ups), WARN for potential challenges that don’t split the application, Mistake for actual complications, and Deadly once the system can’t go on.
Stay clear of flooding your logs with abnormal or irrelevant information. Too much logging can obscure vital messages and decelerate your program. Concentrate on vital functions, state variations, input/output values, and critical final decision details within your code.
Structure your log messages Obviously and consistently. Include things like context, including timestamps, ask for IDs, and function names, so it’s much easier to trace difficulties in distributed units or multi-threaded environments. Structured logging (e.g., JSON logs) might make it even much easier to parse and filter logs programmatically.
For the duration of debugging, logs let you monitor how variables evolve, what conditions are satisfied, and what branches of logic are executed—all without halting This system. They’re Particularly precious in manufacturing environments wherever stepping by means of code isn’t probable.
Moreover, use logging frameworks and applications (like Log4j, Winston, or Python’s logging module) that help log rotation, filtering, and integration with monitoring dashboards.
Eventually, intelligent logging is about balance and clarity. By using a well-believed-out logging tactic, you can decrease the time it will require to spot difficulties, gain deeper visibility into your programs, and Enhance the In general maintainability and reliability of the code.
Assume Like a Detective
Debugging is not simply a complex endeavor—it's a type of investigation. To properly detect and fix bugs, developers need to approach the process like a detective solving a thriller. This frame of mind helps break down complicated concerns into manageable parts and adhere to clues logically to uncover the basis result in.
Start off by collecting proof. Consider the signs or symptoms of the condition: mistake messages, incorrect output, or efficiency concerns. Similar to a detective surveys a criminal offense scene, acquire as much pertinent details as it is possible to with no jumping to conclusions. Use logs, check scenarios, and consumer studies to piece collectively a clear image of what’s happening.
Next, variety hypotheses. Talk to you: What might be causing this actions? Have any variations not long ago been manufactured to your codebase? Has this situation occurred prior to less than similar instances? The target is usually to narrow down possibilities and detect likely culprits.
Then, check your theories systematically. Try to recreate the condition in a very managed surroundings. If you suspect a selected operate or component, isolate it and confirm if the issue persists. Similar to a detective conducting interviews, request your code questions and Permit the final results direct you nearer to the truth.
Pay back near attention to smaller specifics. Bugs normally conceal in the least predicted places—just like a lacking semicolon, an off-by-just one error, or a race affliction. Be comprehensive and affected individual, resisting the urge to patch The problem with out absolutely knowing it. Non permanent fixes may possibly hide the true trouble, only for it to resurface afterwards.
Finally, continue to keep notes on That which you tried and figured out. Equally as detectives log their investigations, documenting your debugging method can help you save time for potential issues and aid others realize your reasoning.
By imagining similar to a detective, developers can sharpen their analytical capabilities, solution difficulties methodically, and turn into more practical at uncovering concealed problems in intricate devices.
Write Assessments
Crafting tests is among the simplest tips on how to boost your debugging capabilities and Over-all enhancement efficiency. Tests not just assistance catch bugs early but also serve as a safety net that gives you self-assurance when generating improvements on your codebase. A perfectly-analyzed application is easier to debug because it enables you to pinpoint specifically in which and when a difficulty happens.
Start with unit tests, which focus on individual features or modules. These tiny, isolated exams can swiftly reveal whether or not a specific bit of logic is Doing the job as envisioned. Every time a examination fails, you right away know wherever to seem, drastically lowering time spent debugging. Device assessments are Specially beneficial for catching regression bugs—problems that reappear after Beforehand staying preset.
Following, integrate integration checks and conclusion-to-conclude tests into your workflow. These assistance be sure that a variety of elements of your software operate jointly smoothly. They’re significantly valuable for catching bugs that happen in elaborate programs with several factors or companies interacting. If some thing breaks, your checks can let you know which Element of the pipeline unsuccessful and below what disorders.
Composing checks also forces you to Imagine critically about your code. To check a characteristic properly, you may need to know its inputs, predicted outputs, and edge situations. This level of comprehension naturally qualified prospects to raised code framework and much less bugs.
When debugging a problem, producing a failing test that reproduces the bug might be a robust first step. After the check fails continually, you can target correcting the bug and watch your examination go when The difficulty is resolved. This strategy makes certain that the same bug doesn’t return Later on.
Briefly, creating exams turns debugging from the disheartening guessing sport into a structured and predictable approach—serving to you capture much more bugs, more quickly plus much more reliably.
Choose Breaks
When debugging a tough problem, it’s effortless to be immersed in the condition—staring at your screen for hours, attempting Remedy soon after Option. But One of the more underrated debugging applications is simply stepping absent. Having breaks aids you reset your brain, minimize stress, and sometimes see The problem from the new point of view.
When you are also near to the code for also extended, cognitive fatigue sets in. You may begin overlooking clear problems or misreading code that you just wrote just hrs earlier. In this point out, your Mind will become considerably less productive at difficulty-solving. A brief wander, a coffee split, as well as switching to a special job for ten–quarter-hour can refresh your emphasis. Several developers report getting the foundation of a difficulty after they've taken the perfect time check here to disconnect, allowing their subconscious perform from the track record.
Breaks also assist reduce burnout, In particular for the duration of lengthier debugging classes. Sitting down in front of a display screen, mentally stuck, is don't just unproductive and also draining. Stepping away helps you to return with renewed Strength and also a clearer attitude. You may instantly recognize a missing semicolon, a logic flaw, or perhaps a misplaced variable that eluded you right before.
In case you’re stuck, a superb rule of thumb will be to set a timer—debug actively for forty five–sixty minutes, then take a 5–ten moment split. Use that point to move all over, stretch, or do anything unrelated to code. It may come to feel counterintuitive, especially beneath limited deadlines, nevertheless it basically contributes to a lot quicker and simpler debugging in the long run.
In a nutshell, having breaks isn't an indication of weak spot—it’s a smart approach. It presents your brain Room to breathe, increases your perspective, and aids you steer clear of the tunnel eyesight that often blocks your development. Debugging is a mental puzzle, and rest is an element of resolving it.
Discover From Every single Bug
Each individual bug you encounter is much more than simply A short lived setback—It is really an opportunity to expand being a developer. Irrespective of whether it’s a syntax mistake, a logic flaw, or maybe a deep architectural issue, each one can educate you a thing important if you take some time to mirror and examine what went Erroneous.
Get started by inquiring oneself some vital questions once the bug is solved: What brought about it? Why did it go unnoticed? Could it have been caught earlier with much better methods like unit testing, code critiques, or logging? The answers frequently reveal blind spots in your workflow or understanding and help you build stronger coding habits going forward.
Documenting bugs can also be an excellent pattern. Continue to keep a developer journal or manage a log in which you Notice down bugs you’ve encountered, how you solved them, and Everything you learned. Over time, you’ll begin to see designs—recurring concerns or typical mistakes—that you can proactively stay clear of.
In staff environments, sharing Anything you've uncovered from a bug with your friends might be Specifically powerful. Irrespective of whether it’s via a Slack message, a brief publish-up, or a quick awareness-sharing session, supporting Other individuals steer clear of the similar concern boosts team performance and cultivates a more robust Studying society.
A lot more importantly, viewing bugs as lessons shifts your mentality from annoyance to curiosity. As opposed to dreading bugs, you’ll start appreciating them as vital parts of your progress journey. In any case, a lot of the ideal builders will not be those who compose fantastic code, but people who constantly study from their errors.
In the long run, each bug you deal with provides a fresh layer towards your skill established. So future time you squash a bug, take a minute to replicate—you’ll come away a smarter, additional capable developer on account of it.
Summary
Enhancing your debugging capabilities will take time, exercise, and patience — nevertheless the payoff is big. It makes you a more productive, self-assured, and able developer. The next time you are knee-deep in the mysterious bug, try to remember: debugging isn’t a chore — it’s an opportunity to become far better at That which you do.